Vue Js Remove Item from Array by Id: To remove an item from an array in Vue.js based on its ID, you can use the Array.prototype.filter() method to create a new array that excludes the item with the matching ID.
First, you need to locate the index of the item in the array using the Array.prototype.findIndex() method. Once you have the index, you can use Array.prototype.splice() method to remove the item from the array.
Alternatively, you can use the Vue.js built-in $delete() method to remove the item by index or by reference.
Overall, the process involves finding the index of the item to remove, using either Array.prototype.splice() or Vue.js $delete() method to remove the item from the array.
Vue Js remove selected item from a list
How can you remove an item from an array in Vue.js based on its ID property?
This code is a Vue.js application that defines a data object containing an array of items with their respective IDs and names. The app also defines a method called “removeItem” that takes an item’s ID as a parameter and removes the item from the items array if it exists.
In summary, this code creates a simple application that displays a list of items and allows the user to remove items from the list.
Vue Js Remove item from Array by ID Example
<div id="app">
<ul>
<li v-for="item in items" :key="item.id">
{{ item.name }}
<button @click="removeItem(item.id)">Remove</button>
</li>
</ul>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
items: [
{ id: 1, name: 'VUE' },
{ id: 2, name: 'React' },
{ id: 3, name: 'Angular' },
{ id: 4, name: 'PHP' }
]
};
},
methods: {
removeItem(id) {
const index = this.items.findIndex(item => item.id === id)
if (index !== -1) {
this.items.splice(index, 1)
}
}
}
});
app.mount('#app');
</script>
Output of Vue JS Remove item from array by id
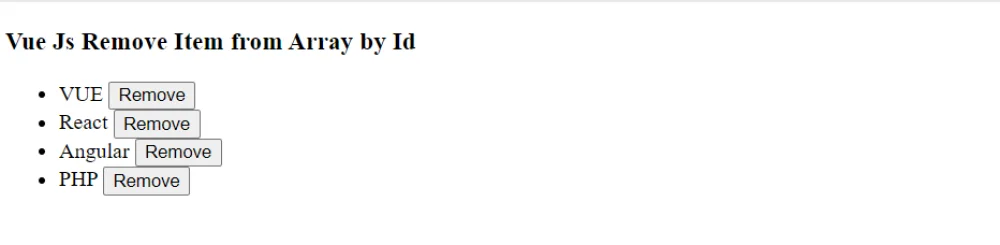